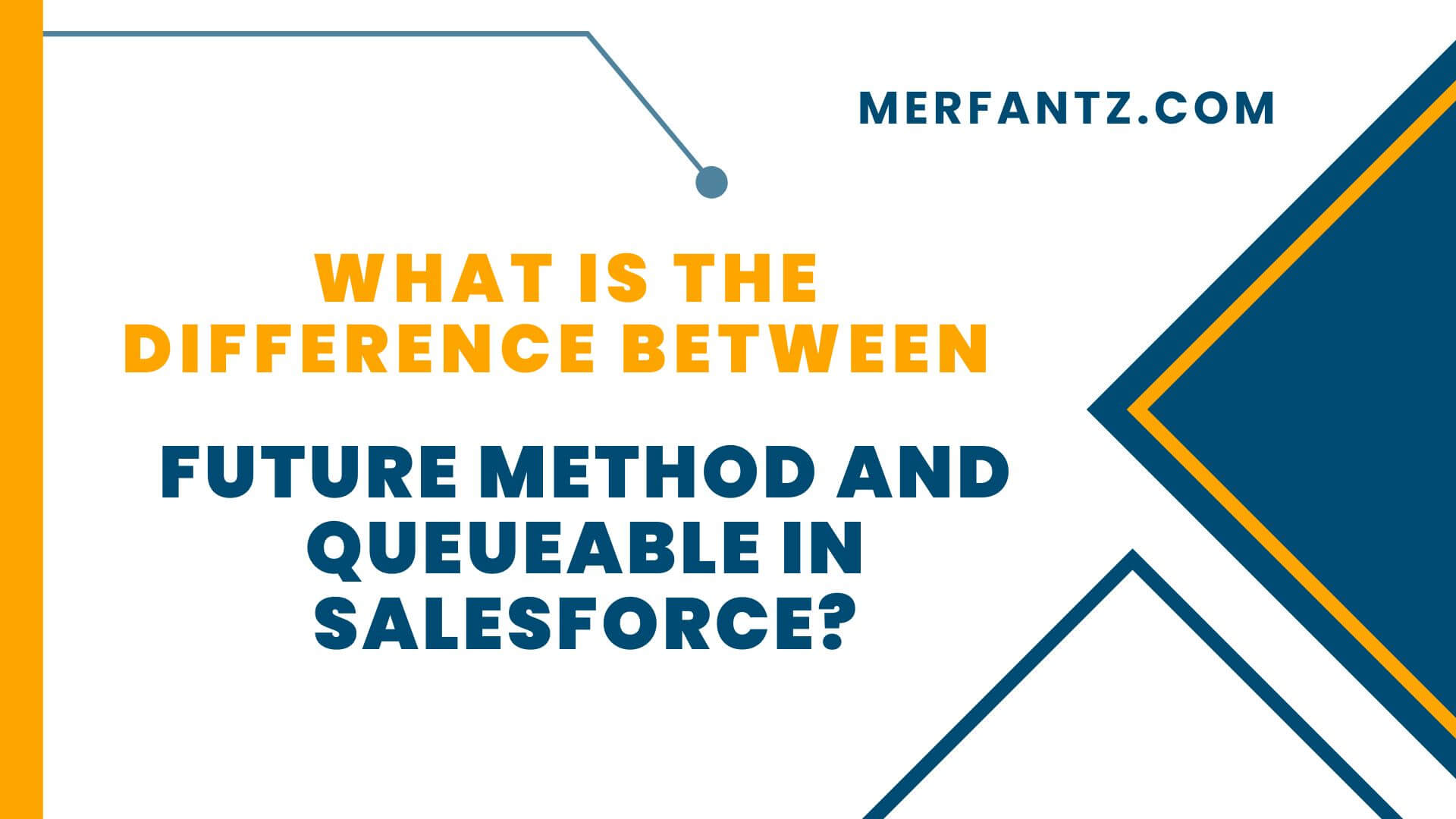
In Salesforce, both Future Methods and Queueable Apex are used to run processes asynchronously. They help to offload time-consuming tasks, ensuring that the user experience remains smooth while complex operations happen in the background. While both serve the purpose of asynchronous execution, they come with distinct features, use cases, and limitations. Let’s dive into the key differences between Future Methods and Queueable Apex.
- Basic Concept
- Future Methods: Introduced to handle asynchronous operations with simple one-off tasks, Future methods are annotated with @future and used to execute processes at a later time.
- Queueable Apex: Queueable Apex offers a more flexible and powerful alternative to Future Methods. It allows chaining of jobs, tracking of job status, and more complex, stateful operations.
- Chaining and Job Management
- Future Methods:
- No Chaining Support: Future methods do not support chaining. You cannot call another future method from within a future method, nor can you manage the sequence of operations.
- Job Monitoring: There’s no direct way to monitor the execution of a future method or its status.
- Queueable Apex:
- Supports Chaining: You can chain jobs in Queueable Apex. Once a job completes, you can initiate another job, making it ideal for complex workflows.
- Job Monitoring: Queueable jobs are tracked as AsyncApexJob objects, allowing you to query and monitor their status.
- State Handling
- Future Methods:
- Stateless: Future methods are stateless, meaning they cannot retain any state between method executions. Only primitive types (like strings, integers) or collections of primitive types are allowed as method parameters.
- Queueable Apex:
- Stateful: Queueable Apex can maintain state between different executions. This means you can pass objects or more complex data structures, making it suitable for tasks that require retaining state over multiple asynchronous calls.
- Error Handling
- Future Methods:
- Limited Error Handling: Future methods do not allow direct error handling, so any exceptions that occur will not bubble up to the calling context. The errors are logged but must be managed through other logging mechanisms.
- Queueable Apex:
- Enhanced Error Handling: Queueable Apex supports try-catch blocks, allowing for more granular error handling and logging within the job itself.
- Execution Limits
- Future Methods:
- Limited Flexibility: Future methods have a limit of 50 future method calls per transaction, and there’s no option to batch or chunk larger processes.
- Queueable Apex:
- Higher Limits: Queueable Apex allows you to submit up to 50 jobs per transaction, but because jobs can chain, you can execute more processes. This offers more flexibility in managing large operations.
- When to Use
- Future Methods:
- Best suited for simple, one-off asynchronous tasks that don’t require chaining, state, or complex error handling.
- Example: Sending an email or making an external API call that doesn’t require any follow-up processing.
- Queueable Apex:
- Ideal for more complex asynchronous tasks where you need job chaining, state management, or error handling.
- Example: When handling large data volumes that need to be processed in stages or need follow-up processing across multiple jobs.
- Code Structure
- Future Method Example:
public class FutureExample {
@future
public static void processFutureMethod(String accountId) {
Account acc = [SELECT Id, Name FROM Account WHERE Id = :accountId];
acc.Name = ‘Updated Name’;
update acc;
}
}
- Queueable Apex Example:
public class QueueableExample implements Queueable {
private String accountId;
public QueueableExample(String accountId) {
this.accountId = accountId;
}
public void execute(QueueableContext context)
{Account acc = [SELECT Id, Name FROM Account WHERE Id = :accountId];
acc.Name = ‘Updated Name’;
update acc;
}
}
- Future Method vs Queueable Summary
Feature | Future Methods | Queueable Apex |
Chaining | Not Supported | Supported |
State Handling | Stateless | Stateful |
Error Handling | Limited | Enhanced |
Monitoring | Not Available | Job Status Available |
Use Case | Simple Asynchronous Tasks | Complex Asynchronous Tasks |
Limits | 50 Jobs per Transaction | 50 Jobs per Transaction + Chaining |
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
While Future Methods offer a simple and quick solution for asynchronous processing, Queueable Apex is a more robust and flexible option that allows for job chaining, state management, and better error handling. If you’re dealing with complex business logic that requires multiple steps or tracking, Queueable Apex is the way to go. However, if you’re looking for a quick way to offload simple tasks, Future Methods can get the job done.