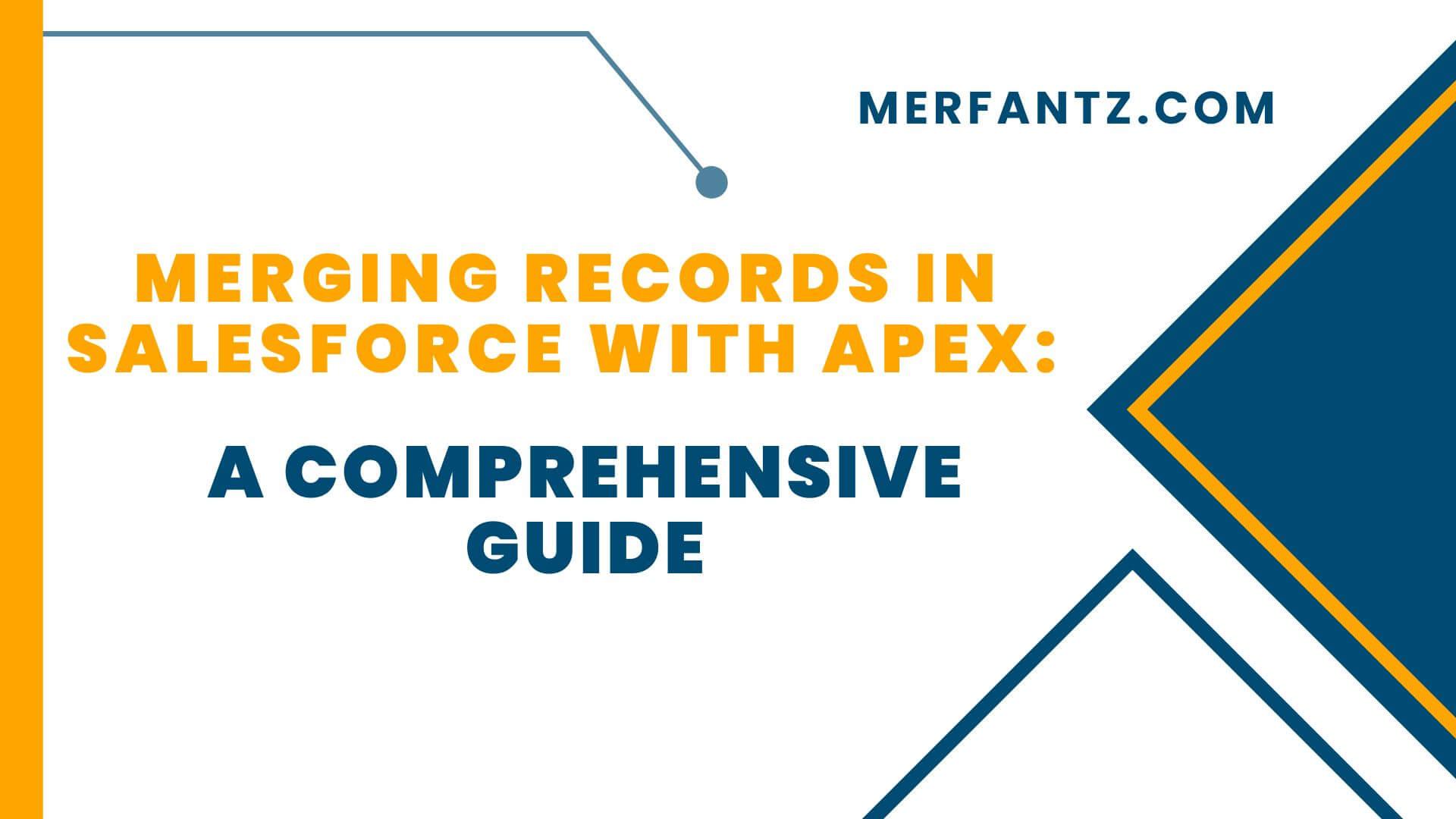
In Salesforce, managing duplicate records such as Leads, Contacts, Cases, or Accounts is essential for maintaining data integrity and streamlining business processes. Duplicate records can lead to confusion, complicate data management, and reduce organizational efficiency. To address this issue, Salesforce offers two main methods for merging records in Apex: the merge statement and the Database.merge() method.
This guide will explore both methods in detail, providing examples and insights on how to effectively merge records in Salesforce using Apex.
Why Merging Records is Crucial
Merging records is vital for consolidating data into a single “master” record. This process automatically deletes duplicates while preserving relationships with related records. For example, when merging duplicate Account records, Salesforce will reassign related Contacts to the master Account, ensuring no data is lost.
Approaches to Merging Records in Apex
Salesforce provides two primary approaches to merge records:
- Using the merge statement
- Using the Database.merge() method
Approach 1: Using the Apex merge Statement
The merge statement offers a straightforward approach to merging records. It allows you to combine up to three records of the same object type, automatically deleting duplicate records and ensuring that related records (such as Contacts or Opportunities) are reassigned to the master record.
Example: Merging Two Account Records
Here’s an example of how to merge two Account records:
apex code :
public class AccountMergeExample {
public static void mergeAccountsExample(Account masterAccount, Account duplicateAccount) {
try {
// Merge the two accounts, keeping ‘masterAccount’ as the master record
merge masterAccount duplicateAccount;
System.debug(‘Merge successful. Master Account ID: ‘ + masterAccount.Id);
} catch (DmlException e) {
System.debug(‘An error occurred while merging accounts: ‘ + e.getMessage());
}
}
}
Test Class for Account Merge
In Salesforce, it’s a best practice to create test classes to validate the functionality of your merge operations. Below is a test class to verify the merge logic:
apex code :
@isTest
public class AccountMergeExampleTest {
@isTest
static void testMergeAccounts() {
List<Account> accList = new List<Account>{
new Account(Name=’Master Account’),
new Account(Name=’Duplicate Account’)
};
insert accList;
Account masterAcct = [SELECT Id, Name FROM Account WHERE Name = ‘Master Account’ LIMIT 1];
Account mergeAcct = [SELECT Id, Name FROM Account WHERE Name = ‘Duplicate Account’ LIMIT 1];
// Create a related Contact and associate it with the duplicate account
Contact con = new Contact(FirstName=’Joe’, LastName=’Merged’, AccountId=mergeAcct.Id);
insert con;
// Merge the accounts
AccountMergeExample.mergeAccountsExample(masterAcct, mergeAcct);
// Verify that the Contact was reparented to the master account
masterAcct = [SELECT Id, Name, (SELECT Id, LastName FROM Contacts) FROM Account WHERE Id = :masterAcct.Id];
System.assert(masterAcct.Contacts.size() > 0);
System.assertEquals(‘Merged’, masterAcct.Contacts[0].LastName);
// Verify that the duplicate account was deleted
Account[] result = [SELECT Id FROM Account WHERE Id = :mergeAcct.Id];
System.assertEquals(0, result.size());
}
}
How It Works:
- The master Account is retained, while the duplicate Account is merged into it.
- Any related records (like Contacts) from the duplicate Account are reassigned to the master Account.
- After the merge, the duplicate Account is deleted, and we confirm that the related Contact was reassigned correctly.
Approach 2: Using the Database.merge() Method
The Database.merge() method offers more control over the merging process. This method is particularly useful when you need to handle errors gracefully without stopping execution. Unlike the merge statement, which throws exceptions when errors occur, Database.merge() returns a MergeResult object that provides details on success and error messages.
Example: Merging Accounts with Database.merge()
Here’s an example of how to merge multiple accounts using the Database.merge() method:
apex code :
public class AccountMergeExample {
public static void mergeDuplicateAccounts() {
// Create master account
Account master = new Account(Name=’Account1′);
insert master;
// Create duplicate accounts
Account[] duplicates = new Account[]{
new Account(Name=’Account1, Inc.’),
new Account(Name=’Account 1′)
};
insert duplicates;
// Create a child contact and associate it with the first duplicate account
Contact c = new Contact(FirstName=’Joe’, LastName=’Smith’, AccountId=duplicates[0].Id);
insert c;
// Merge accounts into master
Database.MergeResult[] results = Database.merge(master, duplicates, false);
for (Database.MergeResult res : results) {
if (res.isSuccess()) {
// Log merged records and reparented related records
List<Id> mergedIds = res.getMergedRecordIds();
System.debug(‘IDs of merged records: ‘ + mergedIds);
// Log the ID of the reparented record (the contact ID)
System.debug(‘Reparented record ID: ‘ + res.getUpdatedRelatedIds());
} else {
for (Database.Error err : res.getErrors()) {
System.debug(err.getMessage());
}
}
}
}
}
How It Works:
- First, we create a master Account and two duplicate Accounts.
- A Contact is associated with the first duplicate Account.
- The Database.merge() method merges the duplicate Accounts into the master Account, and any related records (like Contacts) are automatically reassigned.
- Any errors encountered during the merge process are captured and logged without interrupting the execution.
Comparison: merge vs. Database.merge()
Feature | merge Statement | Database.merge() Method |
Error Handling | Throws exceptions directly | Returns errors in a MergeResult object |
Use Case | Simple merges with minimal custom logic | More control and advanced error handling |
Graceful Execution | Stops execution if an error occurs | Allows error handling without stopping execution |
Bulk Operations | Merges up to three records | Merges up to three records |
Best Practices for Merging Records
- Error Handling: If you need advanced control or custom error handling, Database.merge() is the better choice. It allows you to capture errors and continue execution without interruptions.
- Backup Your Data: Always back up your data before performing merge operations to prevent accidental data loss.
- Test Your Merges: Ensure your merge logic is thoroughly tested, especially when dealing with related records.
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Merging records in Salesforce using Apex is an essential technique to maintain clean, accurate data. Whether you choose the merge statement or the Database.merge() method depends on your specific requirements, such as error handling and control. Both methods allow you to consolidate records and reassign related data, but Database.merge() provides more flexibility for complex use cases. Always test your merge logic to avoid data issues and ensure smooth execution.
By following these best practices, you can confidently merge records in Salesforce and maintain a high standard of data integrity.