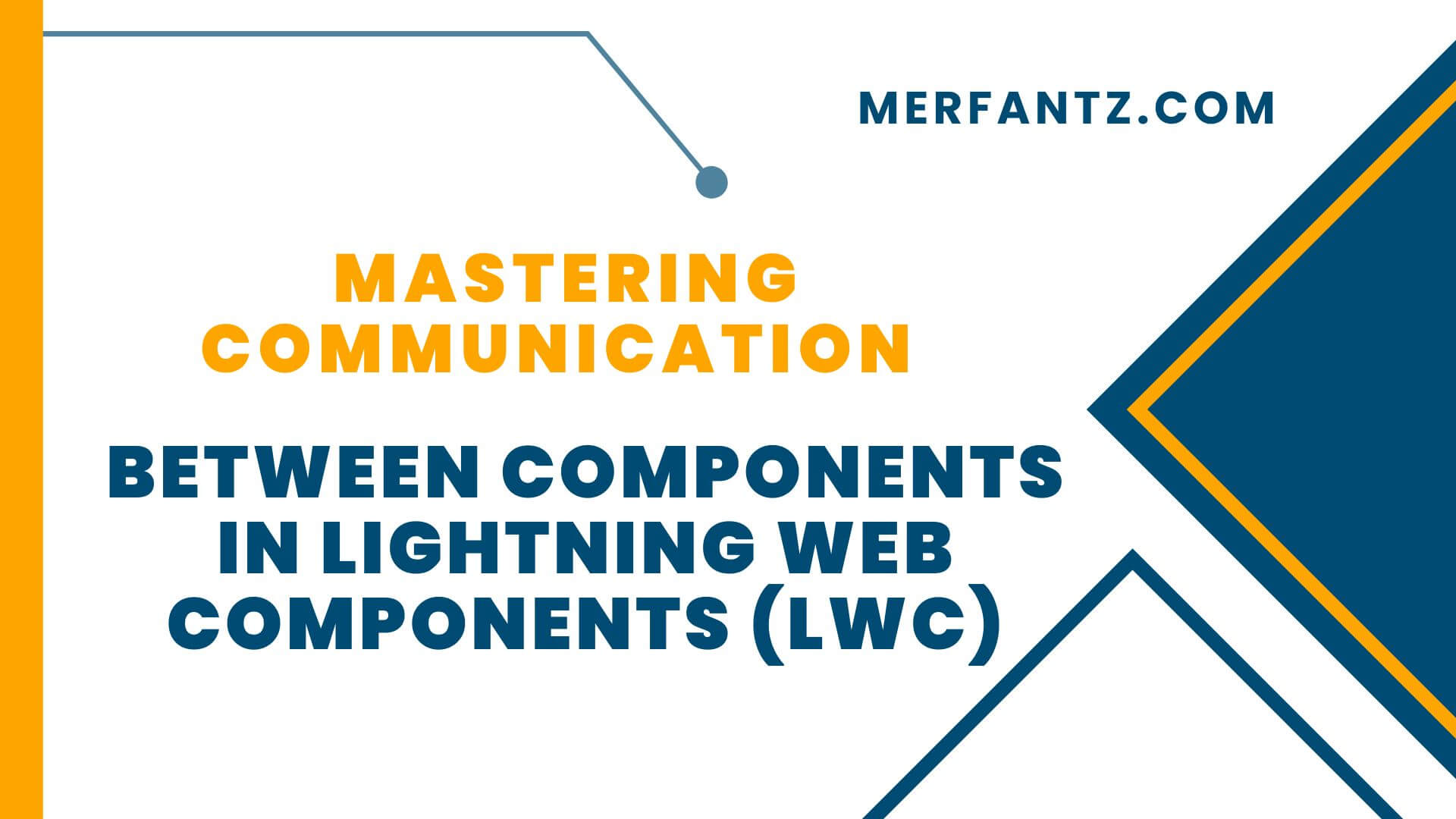
Introduction:
In Salesforce development, seamless communication between components is critical for building scalable applications. Lightning Web Components (LWC) provide robust mechanisms for interaction between parent and child components. This blog explores both Parent-to-Child and Child-to-Parent Communication, along with practical examples and best practices.
What is Component Communication?
Component communication refers to the ability of one component to send data or trigger actions in another.
Parent-to-Child Communication: A parent component passes data or triggers actions in its child components.
Child-to-Parent Communication: A child component notifies or sends data back to its parent component using events.
Parent-to-Child Communication
- Passing Data Using Properties
Parent components can pass data to child components by binding values to @api properties in the child.
Example:
Child Component (childComponent.js)
import { LightningElement, api } from ‘lwc’;
export default class ChildComponent extends LightningElement {
@api message; // Exposed to parent
}
Child Component (childComponent.html)
<template>
<p>Message from Parent: {message}</p>
</template>
Parent Component (parentComponent.html)
<template>
<c-child-component message=”Hello from Parent!”></c-child-component>
</template>
- Invoking Child Methods
A parent component can call methods defined in the child using the @api decorator.
Example:
Child Component (childComponent.js)
import { LightningElement, api } from ‘lwc’;
export default class ChildComponent extends LightningElement {
@api showMessage() {
alert(‘This is a message from the child component!’);
}
}
Parent Component (parentComponent.js)
import { LightningElement } from ‘lwc’;
export default class ParentComponent extends LightningElement {
handleButtonClick() {
this.template.querySelector(‘c-child-component’).showMessage();
}
}
Parent Component (parentComponent.html)
<template>
<lightning-button label=”Call Child Method” onclick={handleButtonClick}></lightning-button>
<c-child-component></c-child-component>
</template>
Child-to-Parent Communication
Using Custom Events
A child component can communicate with its parent by dispatching custom events. The parent listens for these events and handles them as needed.
Example:
Child Component (childComponent.js)
import { LightningElement } from ‘lwc’;
export default class ChildComponent extends LightningElement {
handleButtonClick() {
const event = new CustomEvent(‘notify’, {
detail: { message: ‘Hello from Child!’ }
});
this.dispatchEvent(event); // Notify the parent
}
}
Child Component (childComponent.html)
<template>
<lightning-button label=”Notify Parent” onclick={handleButtonClick}></lightning-button>
</template>
Parent Component (parentComponent.js)
import { LightningElement } from ‘lwc’;
export default class ParentComponent extends LightningElement {
handleNotification(event) {
alert(`Received message: ${event.detail.message}`);
}
}
Parent Component (parentComponent.html)
<template>
<c-child-component onnotify={handleNotification}></c-child-component>
</template>
Best Practices for Component Communication
- Keep Components Decoupled:
Use generic properties and event names to maintain reusability. - Avoid Overusing API Methods:
Use property binding for data transfer and reserve method invocation for actions.
- Use Event Detail for Data Transfer:
Always use the detail property in custom events to pass structured data.
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Understanding both Parent-to-Child and Child-to-Parent Communication is vital for building robust and interactive Salesforce applications with Lightning Web Components. Using these techniques effectively ensures modularity, reusability, and clarity in your components.