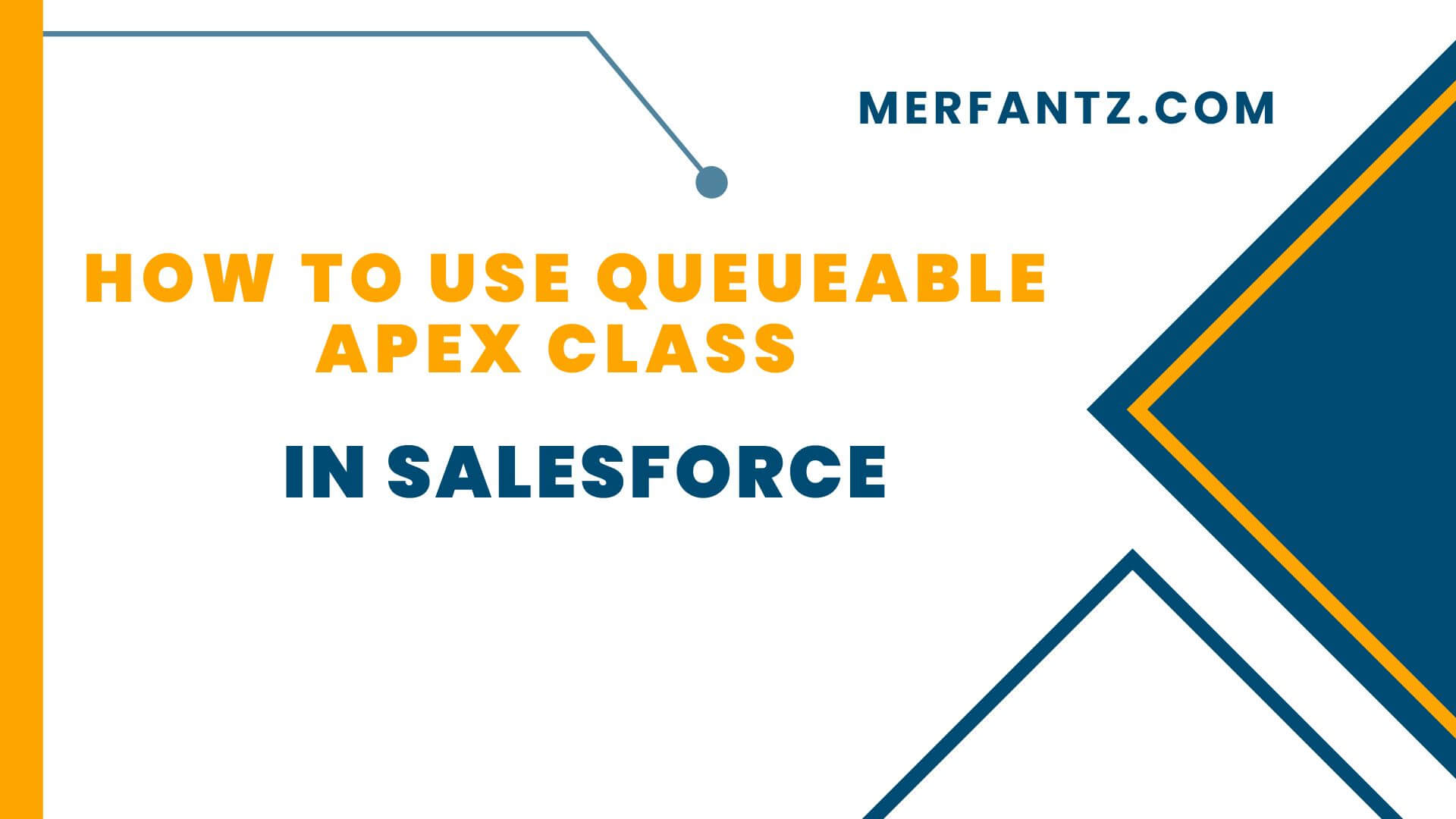
Queueable Apex is a powerful way to run asynchronous processes in Salesforce, providing more flexibility than future methods and allowing complex job chaining. In this guide, we’ll walk through creating and using a Queueable Apex class.
What is Queueable Apex?
Queueable Apex in Salesforce allows you to:
- Run processes asynchronously.
- Chain jobs by adding a new job within the `execute()` method.
- Handle complex data types like objects and custom data structures.
When to Use Queueable Apex?
- When you need to perform an operation that doesn’t need to be completed immediately.
- When you need to chain jobs (running one job after another).
- When you want more complex error handling and monitoring than future methods provide.
Advantages of Queueable Apex
- Chaining Jobs: Unlike future methods, Queueable Apex allows you to chain jobs, meaning you can execute a job and then enqueue another job once the current job completes.
- Flexible Data Types: Queueable Apex supports complex data types, including sObjects and custom objects, making it more versatile for handling various operations.
- Improved Monitoring: Queueable jobs are easier to monitor and manage through the Salesforce UI, allowing you to track their status and view logs for completed or failed jobs.
- Error Handling: Provides enhanced error handling compared to future methods, allowing you to catch and handle exceptions more effectively.
- Job ID Tracking: You can get the job ID when enqueuing a job, enabling you to track the job programmatically if needed.
- Governor Limit Handling: Queueable Apex has higher governor limits for some operations, such as higher heap size limits compared to future methods, making it more efficient for larger data sets.
Creating a Queueable Apex Class
Step 1: Define the Queueable Class
To create a Queueable class, implement the `Queueable` interface in your Apex class. Here’s an example:
public class MyQueueableClass implements Queueable {
public String recordId;
// Constructor to pass parameters to the Queueable class
public MyQueueableClass(String recordId) {
this.recordId = recordId;
}
// The execute method that performs the job
public void execute(QueueableContext context) {
// Fetch the record using the ID
Account acc = [SELECT Id, Name FROM Account WHERE Id = :recordId];
// Perform some operation
acc.Name = ‘Updated Account Name’;
update acc;
}
}
Step 2: Enqueue the Job
To run the Queueable job, use the `System.enqueueJob` method. You can call this from a trigger, another class, or anonymous Apex.
// Enqueue the job
MyQueueableClass job = new MyQueueableClass(‘0015g00000VxxZ2AAJ’);
System.enqueueJob(job);
Advanced Features of Queueable Apex
- Chaining Jobs
You can chain Queueable jobs by enqueuing another job within the `execute()` method.
public void execute(QueueableContext context) {
// Perform some operation
// Chain another Queueable job
MyQueueableClass newJob = new MyQueueableClass(‘0015g00000VxxZ3AAK’);
System.enqueueJob(newJob);
}
- Monitoring Queueable Jobs
Queueable jobs can be monitored through the Salesforce UI:
- Go to Setup > Jobs > Apex Jobs.
- You can view the job’s status, including completed, failed, and processing jobs.
- Using Complex Data Types
Queueable Apex supports complex data types like sObjects and collections. You can pass custom objects and even JSON strings to the Queueable class.
public class MyQueueableClass implements Queueable {
public List<Account> accounts;
public MyQueueableClass(List<Account> accounts) {
this.accounts = accounts;
}
public void execute(QueueableContext context) {
// Perform operations on the list of accounts
for(Account acc : accounts) {
acc.Name = ‘Updated Name’;
}
update accounts;
}
}
Best Practices
- Avoid Long-Running Jobs: Ensure your Queueable jobs do not exceed the governor limits, especially for SOQL queries and DML operations.
- Error Handling : Implement proper error handling to ensure that any exceptions are caught and logged.
- Chaining Limit: Remember that you can only chain up to 50 jobs in a single transaction.
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Queueable Apex provides a robust framework for running asynchronous operations in Salesforce. It allows more flexibility than future methods and is perfect for complex, chained operations. By using Queueable Apex, you can improve your Salesforce application’s efficiency and performance.