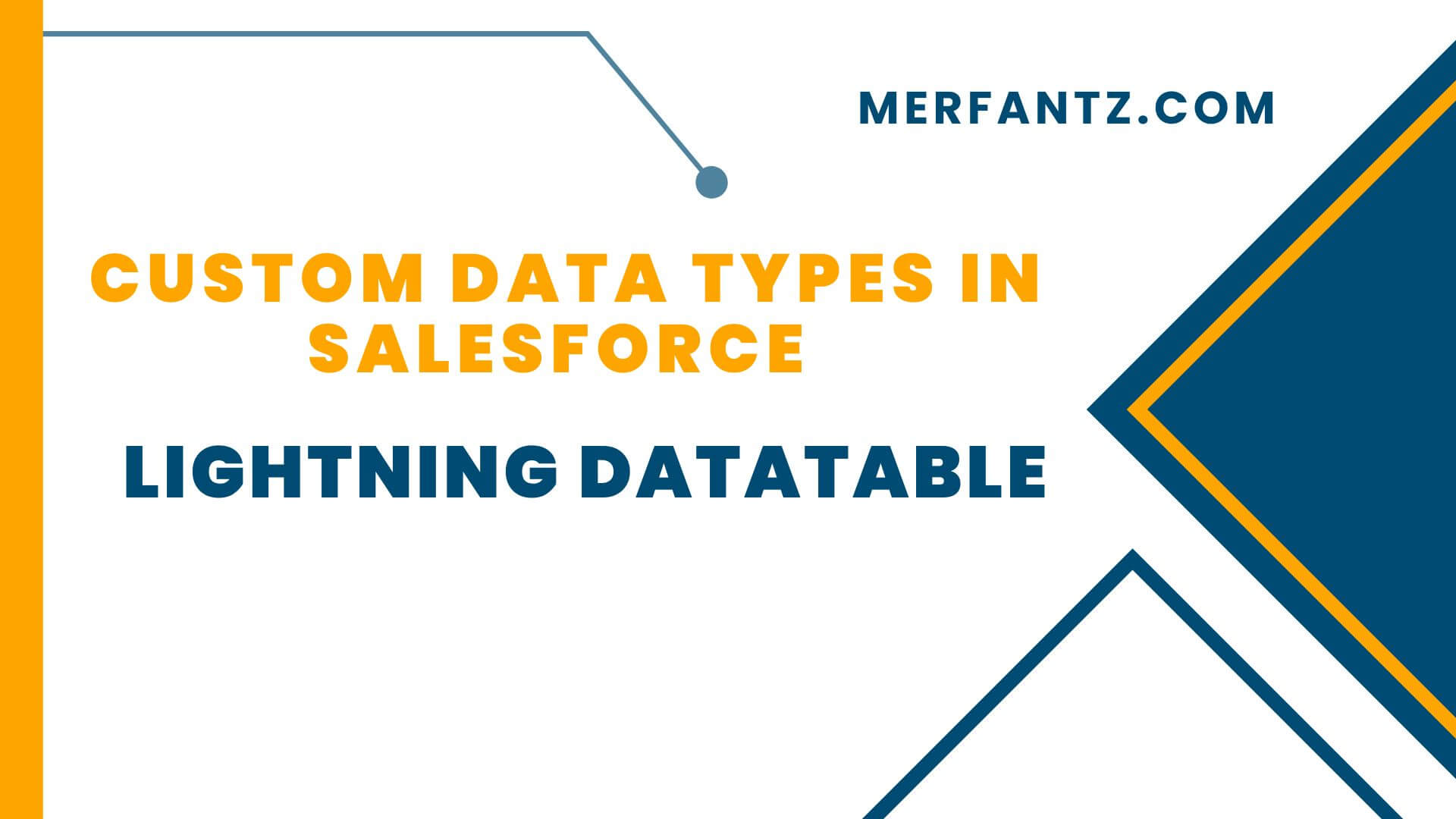
Salesforce’s lightning-datatable component allows developers to display data in a tabular format with built-in support for various standard datatypes. However, sometimes, unique business requirements necessitate the use of custom data types with specialized formatting.
Standard Datatypes Supported by lightning-datatable
The lightning-datatable supports the following standard datatypes:
| action | Boolean | button
| button-icon | currency | date
| date-local | email | location
| number | percent | phone
| text | URL |
Why Custom Data Types?
When the standard datatypes don’t meet specific requirements (e.g., toggle switches or specialized picklists), Salesforce provides the flexibility to extend lightning-datatable with custom datatypes.
Steps to Define Custom Data Types
Step 1: Create a Lightning Component to Extend lightning-datatable
The extended component defines the custom datatypes and their associated templates.
- Custom Component Structure:
customDtTypeLwc/
├── customDtTypeLwc.html
├── customDtTypeLwc.js
├── customDtTypeLwc.js-meta.xml
├── picklistEditable.html
├── picklistNotEditable.html
├── toggelTemplate.html
- Custom Component Files:
HTML Template for Toggle (toggelTemplate.html):
<template>
<c-toggeltype
value={typeAttributes.value}
context={typeAttributes.context}>
</c-toggeltype>
</template>
JavaScript Controller (customDtTypeLwc.js):
import LightningDatatable from ‘lightning/datatable’;
import picklistEditable from ‘./picklistEditable.html’;
import picklistNotEditable from ‘./picklistNotEditable.html’;
import toggelTemplate from ‘./toggelTemplate.html’;
export default class CustomDtTypeLwc extends LightningDatatable {
static customTypes = {
picklistColumn: {
template: picklistNotEditable,
editTemplate: picklistEditable,
typeAttributes: [‘label’, ‘placeholder’, ‘options’, ‘value’, ‘context’],
},
toggel: {
template: toggelTemplate,
typeAttributes: [‘value’, ‘context’],
},
};
}
Step 2: Create a Toggle Lightning Web Component
- HTML (toggeltype.html):
<template>
<div class=”slds-form-element”>
<label class=”slds-checkbox_toggle slds-grid”>
<input
type=”checkbox”
checked={togglevalue}
onchange={handleChange} />
<span class=”slds-checkbox_faux_container”>
<span class=”slds-checkbox_faux”></span>
<span class=”slds-checkbox_on”>Enabled</span>
<span class=”slds-checkbox_off”>Disabled</span>
</span>
</label>
</div>
</template>
- JavaScript (toggeltype.js):
import { LightningElement, api, track } from ‘lwc’;
export default class Toggeltype extends LightningElement {
@api value;
@track togglevalue;
renderedCallback() {
this.togglevalue = this.value;
}
handleChange(event) {
const value = event.target.checked;
this.togglevalue = value;
this.dispatchEvent(new CustomEvent(‘toggelselect’, {
detail: { value },
}));
}
}
Step 3: Define Templates for Picklist
- Read-Only Template (picklistNotEditable.html):
<template>
<span class=”slds-truncate” title={value}>{value}</span>
</template>
- Editable Template (picklistEditable.html):
<template>
<lightning-combobox
label={typeAttributes.label}
value={typeAttributes.value}
options={typeAttributes.options}
placeholder={typeAttributes.placeholder}>
</lightning-combobox>
</template>
Testing the Custom Data Types
Apex Controller
public with sharing class LWCInlineCtrl {
@AuraEnabled(Cacheable = true)
public static List<Contact> getContacts() {
return [
SELECT Id, Name, FirstName, LastName, Phone, Email, GenderIdentity, DoNotCall
FROM Contact
WHERE Email != null AND Phone != null
ORDER BY CreatedDate LIMIT 5
];
}
}
Test Component
- HTML (extended_inline_datatable.html):
<template>
<lightning-card title=”Custom Data Types”>
<template if:true={contacts}>
<c-custom-dt-type-lwc
key-field=”Id”
data={contacts}
columns={columns}>
</c-custom-dt-type-lwc>
</template>
</lightning-card>
</template>
- JavaScript (extended_inline_datatable.js):
import { LightningElement, wire } from ‘lwc’;
import getContacts from ‘@salesforce/apex/LWCInlineCtrl.getContacts’;
const columns = [
{ label: ‘Name’, fieldName: ‘Name’, type: ‘text’ },
{ label: ‘Phone’, fieldName: ‘Phone’, type: ‘phone’ },
{ label: ‘Gender’, fieldName: ‘GenderIdentity’, type: ‘picklistColumn’, editable: true },
{ label: ‘Do Not Call’, fieldName: ‘DoNotCall’, type: ‘toggel’ },
];
export default class Extended_inline_datatable extends LightningElement {
@wire(getContacts) contacts;
columns = columns;
}
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
With custom datatypes, you can meet unique business needs while leveraging the powerful lightning-datatable component. By using custom HTML templates and JavaScript logic, Salesforce provides immense flexibility for data presentation and interaction in Lightning Web Components.