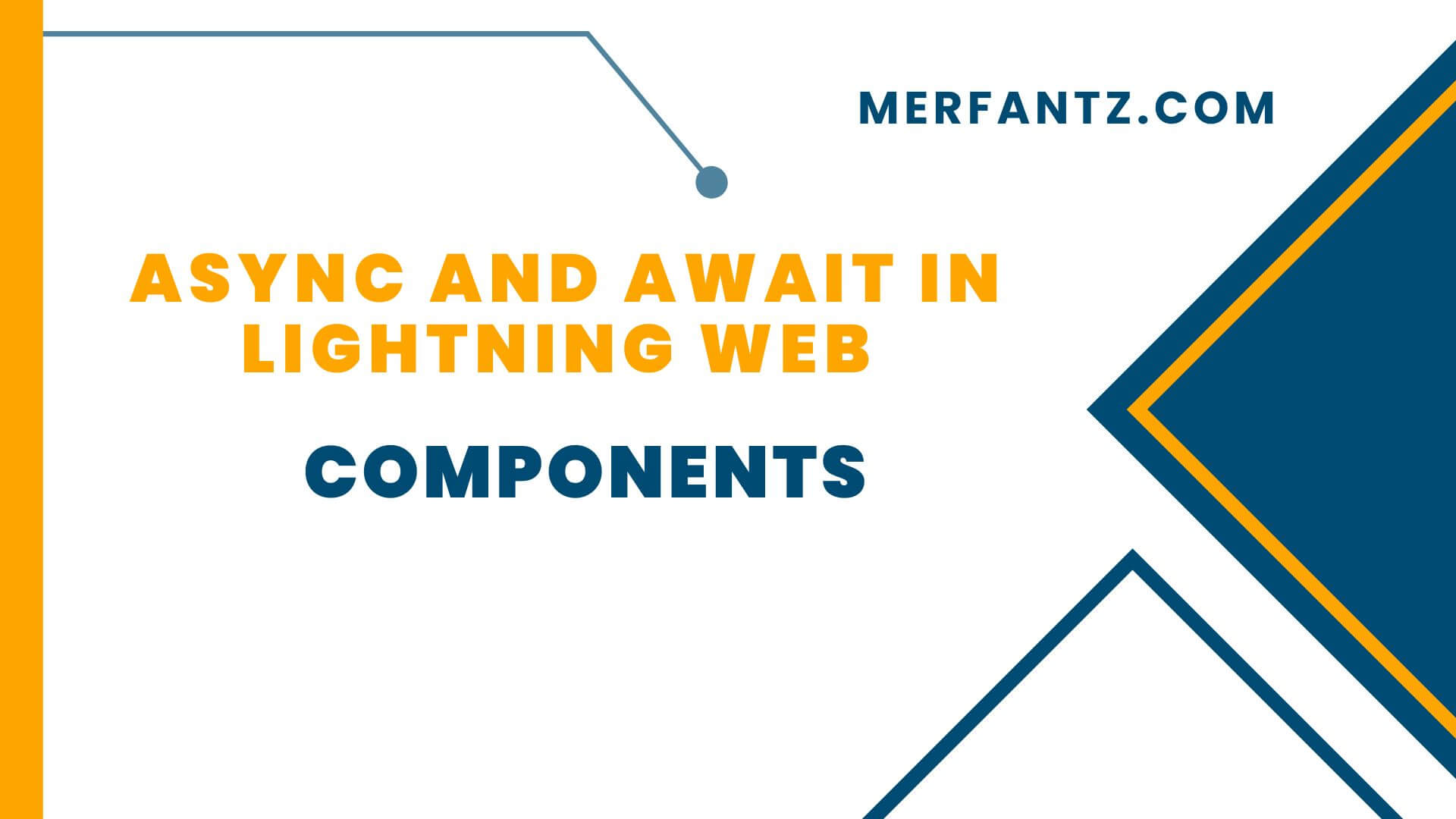
Introduction:
Asynchronous programming is a fundamental aspect of modern web development, allowing developers to create applications that are both efficient and responsive. Managing asynchronous tasks effectively is crucial for maintaining optimal performance and delivering a seamless user experience, particularly in large-scale solutions. In Lightning Web Components (LWC), the async/await syntax provides a straightforward way to handle asynchronous operations. This method enhances code clarity and maintainability, enabling developers to write cleaner and more intuitive logic tailored to real-world needs.
What is Async and Await?
Async/await provides a way to write asynchronous code in JavaScript that resembles synchronous code in both appearance and behavior. It builds upon promises, which handle asynchronous operations.
The async keyword defines an asynchronous function that always returns a promise. Inside this function, the await keyword can be used to pause execution until the associated promise resolves or rejects. This approach makes the code more readable and synchronous-like, as execution appears to halt until the promise is fulfilled.
How to use Async and Await in Lightning Web Components
With a clear understanding of async/await, let’s explore how to apply it in Lightning Web Components. The initial step is to define an asynchronous function. Below is an example of such a function that returns a promise:
async function fetchData() {
const response = await fetch(‘/api/data’);
const data = await response.json();
return data;
}
In this example, we use the fetch API to send a request to an API endpoint. The await keyword pauses the function’s execution until the promise returned by fetch is resolved. Once resolved, we parse the response body as JSON with the response.json() method and then return the resulting data.
To use this function in a Lightning Web Component, we can simply call it from the component’s JavaScript file:
import { LightningElement } from ‘lwc’;
import fetchData from ‘./fetchData’;
export default class MyComponent extends LightningElement {
async connectedCallback() {
const data = await fetchData();
console.log(data);
}
}
Promise with Async and Await
import { LightningElement, api } from ‘lwc’;
import fetchData from ‘@salesforce/apex/MyController.fetchData’;
export default class MyComponent extends LightningElement {
@api recordIds;
data = [];
async connectedCallback() {
const promises = this.recordIds.map(id => fetchData({ recordId: id }));
const results = await Promise.all(promises);
this.data = results;
}
}
Common Use Cases for Async and Await
The use of async and await extends beyond just API calls. They are particularly useful when you need to execute actions that take a considerable amount of time, such as:
- Sending HTTP requests
- Waiting for an asynchronous operation to complete
- Handling multiple promises concurrently
Error Handling with Async and Await
Since async functions return promises, any exception thrown within them results in the rejection of the promise with that exception. This can be managed using the standard try/catch blocks for error handling:
try {
await someAsyncFunction(arg1); // perform the operation
} catch (e) {
// handle the error here
}
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion:
The async/await syntax is a game-changer for asynchronous programming, bringing simplicity and clarity to complex workflows. In Lightning Web Components, this feature enables developers to handle asynchronous tasks like API calls, batch processing, and error handling more effectively. By mastering async/await, you can write cleaner, more maintainable code, ensuring your applications are efficient and user-friendly.
Adopting async/await in your development workflow not only enhances productivity but also lays a strong foundation for building scalable and robust web applications.