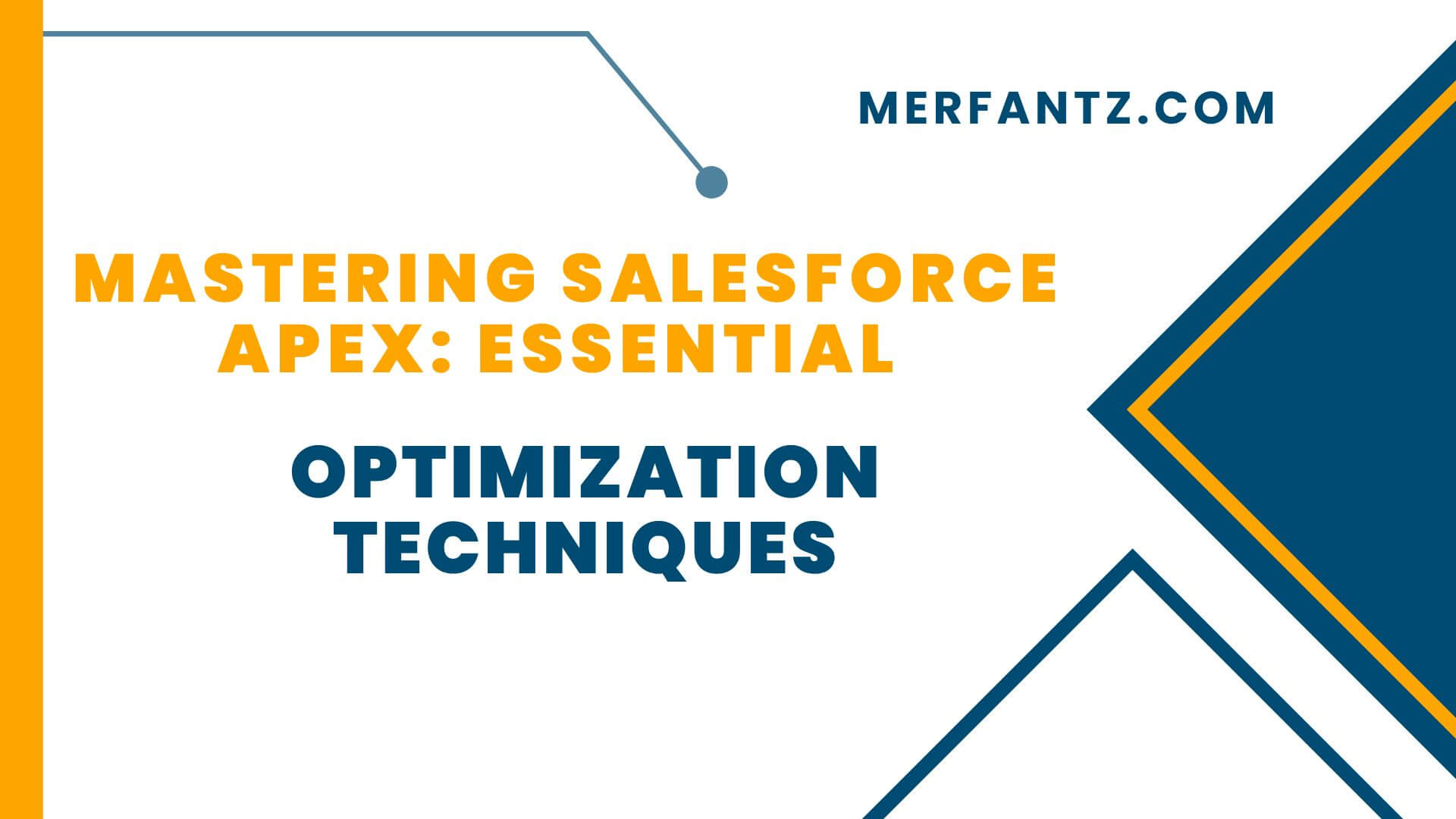
1. The Challenge of Apex Performance
Apex code is the backbone of Salesforce’s customization capabilities, enabling developers to tailor the platform to meet specific business needs. However, ensuring optimal performance in Apex development comes with unique challenges. Salesforce operates on a multi-tenant architecture, meaning resources are shared across multiple customers. To maintain platform stability and fairness, Salesforce enforces governor limits—strict thresholds that developers must respect.
Key Performance Challenges
- Inefficient Processing of Large Data Sets
Apex often processes thousands or even millions of records. Without optimized code, operations such as iterating over large data sets can consume excessive CPU time or memory, causing timeouts or failures. - Excessive Use of SOQL Queries
SOQL (Salesforce Object Query Language) is integral to accessing Salesforce data, but poor query practices—such as querying within loops—can quickly exceed limits.
- Governor Limit Example: Developers are allowed a maximum of 100 SOQL queries per transaction. Exceeding this limit causes a runtime exception.
- Governor Limits in Bulk Data Operations
Apex code is often triggered by bulk operations, such as when importing or updating thousands of records. Poor handling of bulk data can lead to governor limit breaches, particularly for:
- DML (Data Manipulation Language) statements.
- Heap size limits.
- Execution time limits.
2. Bulkification: The Foundation of Apex Optimization
When it comes to efficient Apex development, bulkification stands as the most crucial practice. It ensures that your code can handle multiple records in a single transaction without breaching Salesforce’s governor limits. By adopting bulkification techniques, developers can process large volumes of data efficiently and maintain robust, scalable applications.
What is Bulkification?
Bulkification involves designing your Apex code to handle multiple records simultaneously instead of processing one record at a time. This approach minimizes the number of DML (Data Manipulation Language) statements and SOQL (Salesforce Object Query Language) queries executed, significantly reducing the risk of governor limit errors.
Core Principles of Bulkification
- Bulk DML Operations
Always perform data manipulation (e.g., insert, update, delete) in bulk rather than for individual records.
- Why It Matters: Salesforce imposes a limit of 150 DML statements per transaction. By bulkifying, you can update thousands of records using a single DML operation.
- Avoid SOQL in Loops
Writing SOQL queries inside loops leads to multiple queries being executed—one for each iteration. This can quickly exhaust the limit of 100 SOQL queries per transaction.
- Example: Instead of querying the database for each record, retrieve all necessary data in a single query and process it in memory.
- Leverage Collections
Use collections like lists, sets, and maps to store and process records in bulk. Collections allow you to group data, making it easier to perform bulk operations efficiently.
Non-Bulkified Example
for (Account acc : Trigger.new) {
Account existingAccount = [SELECT Id FROM Account WHERE Id = :acc.Id];
existingAccount.Name = acc.Name;
update existingAccount;
}
Bulkified Example
// Create a list to store records that need updating
List<Account> accountsToUpdate = new List<Account>();
// Iterate through all records in the trigger
for (Account acc : Trigger.new) {
acc.Name = ‘Updated Name’; // Example logic
accountsToUpdate.add(acc);
}
// Perform a single update operation
update accountsToUpdate;
Real-World Application
Scenario:
A developer is tasked with updating 1,000 account records in a single transaction triggered by a batch process. Using non-bulkified code results in a Too Many SOQL Queries error because the SOQL query is executed for every record. By bulkifying the code:
- Only one SOQL query is executed to retrieve all necessary data.
- A single DML statement updates all records simultaneously.
- The transaction completes efficiently without hitting limits.
- Optimizing SOQL Queries
SOQL queries are powerful tools in Apex, but when used incorrectly, they can severely impact performance.
- Avoid SOQL in Loops: Placing SOQL queries inside loops can lead to a rapid increase in execution time and governor limit errors.
Better Practices:
- Query Outside Loops: Fetch data in bulk before the loop starts.
- Use Collections: Store queried data in collections for easy access during processing.
Code Snippet:
Apex Copy code
// Inefficient query
- for (Account acc : Trigger.new) {
- Account existingAccount = [SELECT Id FROM Account WHERE Id = :acc.Id]; // SOQL in loop
- }
// Optimized query
- Set<Id> accountIds = new Set<Id>();
- for (Account acc : Trigger.new) {
- add(acc.Id);
- }
- List<Account> existingAccounts = [SELECT Id FROM Account WHERE Id IN :accountIds]; // Query outside the loop
Indexing for Efficient SOQL: Optimize your queries by indexing fields that are frequently queried.
Example Table:
Field Name | Index Type |
RecordTypeId | Standard Index |
CreatedDate | Standard Index |
AccountNumber | Custom Index
|
4. Efficient Use of Collections in Apex
Collections (lists, maps, and sets) are essential for managing bulk operations effectively. They help reduce the risk of hitting governor limits by allowing you to handle multiple records simultaneously.
Why Use Collections: Collections enable developers to store and manipulate large sets of records efficiently, facilitating bulk operations.
Example Use Case: A developer retrieves multiple related records using maps to optimize data retrieval.
Code Snippet:
Apex Copy code
- // Using a map for efficient retrieval
- Map<Id, Account> accountMap = new Map<Id, Account>([SELECT Id, Name FROM Account WHERE Id IN :accountIds]);
- for (Account acc : Trigger.new) {
- if (accountMap.containsKey(acc.Id)) {
- // Perform operations using accountMap
- }
- }
Visual Needed: Develop a flow diagram showing how data flows into and is processed using collections, illustrating their efficiency.
5. Handling Large Data Volumes (LDV)
As businesses grow, Salesforce developers often face the challenge of managing Large Data Volumes (LDV)—datasets that contain millions of records. Processing LDV in Salesforce requires special attention to performance and scalability, as the platform’s multi-tenant architecture imposes strict resource limits to ensure stability for all users.
Challenges of Working with LDV in Salesforce
Handling LDV can significantly impact system performance, leading to issues such as:
- Query Performance Degradation: Retrieving large datasets without efficient filtering can slow down queries.
- Governor Limit Breaches: Standard Apex transactions struggle with processing LDV due to limits on CPU time, heap size, and DML operations.
- Operational Complexity: Managing updates, deletions, or transformations for millions of records requires robust solutions to avoid errors or partial processing.
Best Practices for Managing LDV
To overcome these challenges, developers should adopt the following strategies:
1. Partition Data
- Break large datasets into smaller, more manageable segments based on criteria such as regions, categories, or date ranges.
- Partitioning helps reduce the number of records processed in a single operation, minimizing the risk of exceeding resource limits.
2. Use Query Filters Efficiently
- Write selective SOQL queries with indexed fields to limit the data returned.
- Example: Instead of querying all accounts, filter by CreatedDate or Industry.
- Use tools like Salesforce Query Optimizer to identify and optimize non-selective queries.
3. Leverage Salesforce’s Bulk API
- The Bulk API is specifically designed for large-scale data operations such as inserting, updating, or deleting millions of records.
- Unlike standard DML operations, the Bulk API processes data asynchronously in chunks, reducing the load on the system.
6. Future Methods, Queueable Apex, and Asynchronous Processing
When synchronous processing fails to meet your performance needs, consider using asynchronous methods, such as Future methods, Queueable Apex, and Batch Apex.
When Synchronous Processing Fails:
- Introduce these concepts to handle larger workloads effectively.
Code Example:
apex
Copy code
- // Future method example
- @future
- public static void updateAccounts(Set<Id> accountIds) {
- List<Account> accountsToUpdate = [SELECT Id FROM Account WHERE Id IN :accountIds];
- for (Account acc : accountsToUpdate) {
- Name = ‘Updated Name’;
- }
- update accountsToUpdate;
- }
Scenario: A developer optimizes a process to update millions of records without hitting limits by leveraging asynchronous processing.
7. Governor Limits: What They Are and How to Avoid Them
Understanding Salesforce’s governor limits is crucial for every developer. These limits ensure the platform remains stable and efficient for all users. Here’s a breakdown of some key governor limits you should be aware of:
Limit Type | Value | Best Practices |
SOQL Queries per Transaction | 100 | Optimize by querying outside loops and using collections. |
DML Statements | 150 | Bulkify your DML operations to minimize calls. |
CPU Time per Transaction | 10,000 ms | Monitor CPU usage and refactor inefficient code. |
Heap Size | 6 MB | Use collections wisely to manage memory effectively. |
Handling Limits Effectively: Use Limits.getQueries() and Limits.getCpuTime() to track your resource consumption. These methods can be integrated into your code to help you stay within the allowed limits.
Example Code Snippet:
Apex Copy code
System.debug(‘SOQL Queries Used: ‘ + Limits.getQueries());
System.debug(‘CPU Time Used: ‘ + Limits.getCpuTime());
This proactive approach will help you avoid unexpected governor limit errors that could impact your application’s performance.
8. Caching and Reducing Repeated Calculations
Optimizing performance through caching is a powerful technique that can significantly enhance the efficiency of your Apex code. By storing frequently accessed data, developers can reduce load times and avoid repeated calculations.
Optimizing Performance with Caching:
- Use Platform Cache to store data temporarily and reduce the need for repeated SOQL queries.
- Implement custom caching solutions using custom objects to hold frequently accessed data.
Code Example:
Apex Copy code
// Implementing a simple cache
Map<Id, Account> accountCache = new Map<Id, Account>();
public Account getAccount(Id accountId) {
if (!accountCache.containsKey(accountId)) {
accountCache.put(accountId, [SELECT Id, Name FROM Account WHERE Id = :accountId]);
}
return accountCache.get(accountId);
}
This caching strategy minimizes the number of SOQL queries, improving performance while managing governor limits effectively.
9. Tools for Debugging and Performance Tuning
Optimizing Apex code requires not just good coding practices but also the ability to identify bottlenecks and inefficiencies. Salesforce provides a robust set of tools for debugging and performance tuning, and there are also external solutions to complement these native capabilities. Leveraging these tools helps developers pinpoint issues, monitor resource usage, and fine-tune their code for maximum efficiency.
Using Salesforce Developer Console
The Salesforce Developer Console is an indispensable tool for debugging and monitoring performance within the Salesforce platform. It provides detailed insights into code execution, resource consumption, and query performance. Here’s how to effectively use the Developer Console for Apex optimization:
1. Debug Logs
- What They Are: Logs that capture detailed information about code execution, including variable values, system events, and resource usage.
- How to Use:
- Open the Developer Console and navigate to Debug → Change Log Levels.
- Set appropriate log levels (e.g., FINEST for detailed logs).
- Execute your code and review the logs under the Logs
- What to Look For:
- Execution time for various operations.
- Governor limit usage (e.g., SOQL queries, DML operations).
- Error messages or unexpected behaviors.
2. SOQL Query Performance
- Why It Matters: Inefficient SOQL queries can drastically impact performance, especially when working with large datasets.
- How to Analyze Queries:
- Use the Query Plan Tool in the Developer Console to evaluate the cost and performance of your SOQL queries.
- Look for high “Cost” values or warnings about non-selective filters.
- Optimization Tips:
- Use indexed fields in WHERE clauses.
- Avoid fetching unnecessary fields.
- Use LIMIT clauses to minimize data retrieval.
3. Execution Overview
- What It Shows: A visual breakdown of resource usage for Apex transactions.
- How to Use:
- Run your Apex code and open the Execution Overview
- Analyze CPU time, heap size, and DML/SOQL operations for each method or class.
- Benefits: Identify specific methods or operations consuming the most resources, allowing you to focus your optimization efforts.
External Tools for Advanced Debugging and Optimization
While the Developer Console is powerful, external tools can provide additional capabilities for query optimization and performance analysis.
1. EverSQL
- What It Does:
- An advanced query optimization tool designed to analyze and improve SOQL (or SQL) queries.
- How It Helps Salesforce Developers:
- Suggests optimized query structures.
- Provides insights into index usage and potential performance improvements.
- Reduces execution time by identifying inefficient patterns.
2. PMD (Programming Mistake Detector)
- What It Does:
- A static code analysis tool that scans Apex code for performance issues, best practice violations, and potential bugs.
- Key Features for Salesforce Developers:
- Detects unnecessary loops or inefficient queries.
- Flags violations of Salesforce best practices.
- Provides actionable recommendations for optimization.
3. Salesforce CLI (Command Line Interface)
- How It Supports Debugging:
- Offers scripting capabilities to automate performance monitoring.
- Provides access to detailed logs and metadata for advanced debugging.
10. Testing for Performance
Why Testing Matters: Regularly testing your Apex code for performance issues before deployment is critical to maintaining a robust application.
Best Practices:
- Run tests with various datasets, especially when dealing with bulk transactions, to identify potential performance bottlenecks.
- Utilize startTest() and Test.stopTest() methods to simulate and measure performance effectively.
Code Example:
apex
Copy code
@isTest
private class PerformanceTest {
static testMethod void testBulkUpdate() {
// Setup test data
List<Account> accounts = new List<Account>();
for (Integer i = 0; i < 10000; i++) {
accounts.add(new Account(Name=’Test Account ‘ + i));
}
insert accounts;
Test.startTest();
// Call your bulk update method here
Test.stopTest();
// Validate results
System.assertEquals(10000, [SELECT COUNT() FROM Account]);
}
}
This approach ensures that your application remains efficient, even as data volume grows.
11. Final Tips for Apex Optimization
Summary of Best Practices: To help solidify your understanding, here’s a quick recap of the key takeaways for optimizing Apex performance:
- Bulkification is Key: Always design your code to handle multiple records in bulk.
- Optimize SOQL Queries: Avoid SOQL in loops and leverage collections.
- Use Collections Wisely: Make use of lists, maps, and sets to manage data effectively.
- Handle Governor Limits Proactively: Monitor your resource usage to stay within limits.
- Test and Monitor Regularly: Validate your code’s performance under different scenarios.
- Leverage Asynchronous Processing: Utilize Future methods, Queueable Apex, and Batch Apex for larger workloads.
12. Call to Action
Engage with the Community: We’d love to hear your thoughts! Share your own optimization challenges and solutions in the comments below.
Join Us: For more in-depth discussions and sharing of insights, consider subscribing to the Salesforce Knowledge Sharing group. Connect with fellow Salesforce developers and stay updated on the latest tips and best practices!