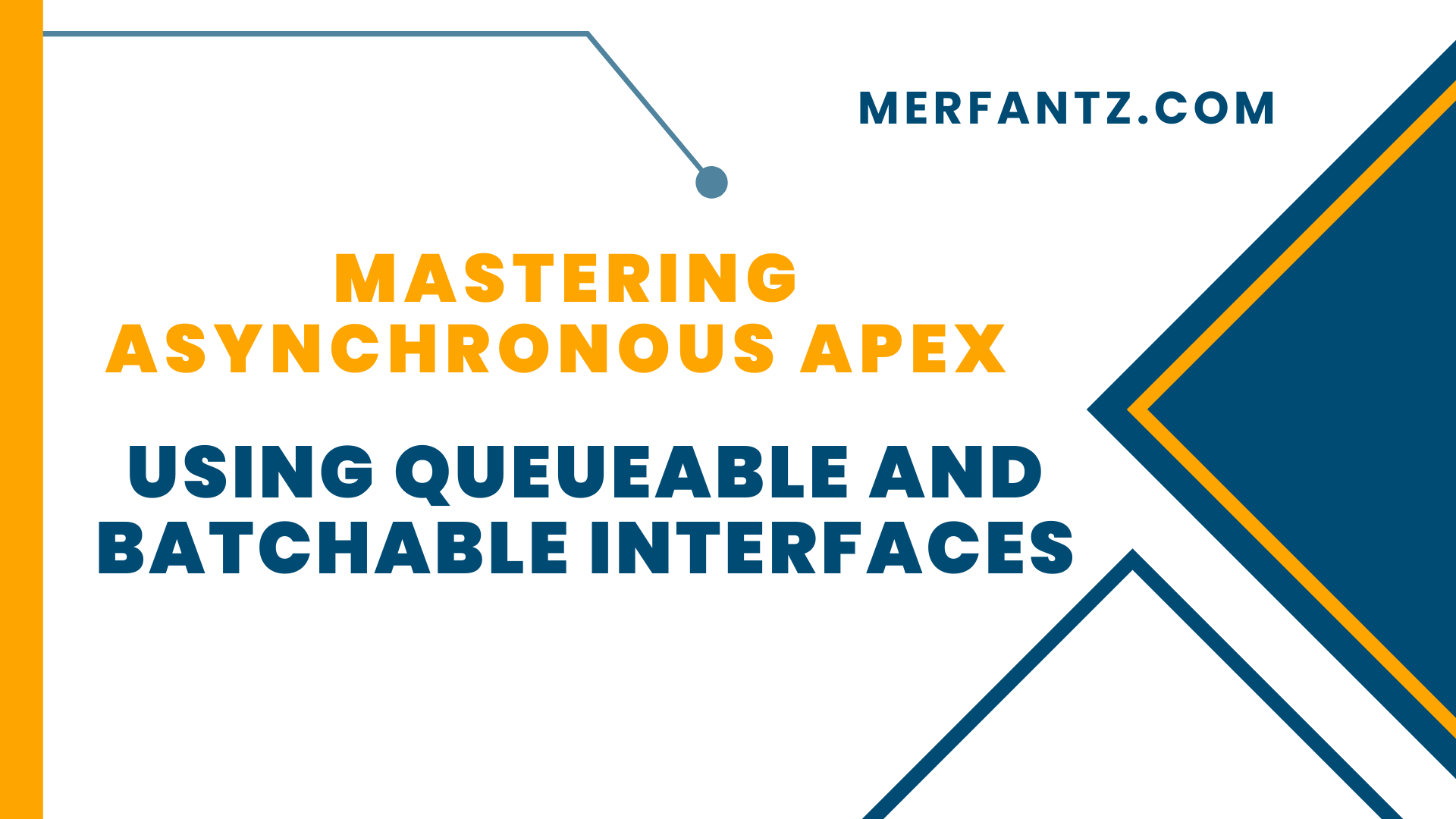
Introduction
Apex is Salesforce’s proprietary programming language, allowing developers to execute flow and transaction control statements on the Salesforce platform’s server. One of the powerful features of Apex is its support for interfaces. Interfaces are a crucial part of object-oriented programming, promoting cleaner, more maintainable code. This blog post will explore various interfaces in Apex, their purpose, and how to implement them effectively in your Salesforce applications.
What are Interfaces?
An interface in Apex defines a contract that classes must adhere to. Unlike classes, interfaces cannot contain any implementation details—only method signatures. This means that any class implementing an interface must provide the specific functionality for all the methods declared in that interface. The primary benefits of using interfaces are:
- Abstraction: They hide the implementation details, allowing for more flexible code.
- Loose Coupling: Classes can interact through interfaces without knowing the specifics of each other’s implementations.
- Reusability: Interfaces allow multiple classes to implement the same methods in different ways.
Commonly Used Interfaces in Salesforce Apex
1. Database.Batchable
Purpose: The Database.Batchable interface is utilized for batch processing, enabling the processing of large data sets asynchronously in manageable chunks.
Implementation: To implement a batch class, you must define three methods: start, execute, and finish.
Example:
global class AccountBatch implements Database.Batchable<SObject> {
global Database.QueryLocator start(Database.BatchableContext BC) {
// Query to select records
return Database.getQueryLocator(‘SELECT Id, Name FROM Account’);
}
global void execute(Database.BatchableContext BC, List<SObject> scope) {
// Process each batch of accounts
for (SObject s : scope) {
Account acc = (Account) s;
acc.Name = acc.Name + ‘ – Processed’; // Example modification
}
update scope; // Update records in the database
}
global void finish(Database.BatchableContext BC) {
// Logic to run after all batches are processed
System.debug(‘Batch processing complete.’);
}
}
2. Iterable
Purpose: The Iterable interface is used to create custom collections that can be iterated over using for loops. This can simplify code when dealing with collections of objects.
Implementation: You need to implement the iterator method that returns an instance of Iterator.
public class NumberCollection implements Iterable<Integer> {
public Integer[] numbers;
public NumberCollection(Integer[] nums) {
this.numbers = nums;
}
public Iterator<Integer> iterator() {
return new NumberIterator();
}
private class NumberIterator implements Iterator<Integer> {
private Integer currentIndex = 0;
public Boolean hasNext() {
return currentIndex < numbers.size();
}
public Integer next() {
return numbers[currentIndex++];
}
}
}
// Usage
NumberCollection collection = new NumberCollection(new Integer[]{1, 2, 3, 4});
for (Integer num : collection) {
System.debug(num);
}
3. Schedulable
Purpose: The Schedulable interface allows developers to run Apex classes at specific times, making it ideal for time-based operations such as scheduled jobs.
Implementation: A class that implements the Schedulable interface must provide the execute method.
Example:
global class ScheduledJob implements Schedulable {
global void execute(SchedulableContext SC) {
// Logic to execute on schedule
System.debug(‘Scheduled job is running!’);
}
}
// Scheduling the job (to be done in Developer Console or another class)
// String cronExpression = ‘0 0 * * * ?’; // Runs every hour
// System.schedule(‘HourlyJob’, cronExpression, new ScheduledJob());
4. Queueable
Purpose: The Queueable interface is used for asynchronous processing, allowing developers to chain jobs and execute them in the background without locking the UI.
Implementation: You can implement the Queueable interface by defining the execute method.
Example:
public class MyQueueableJob implements Queueable {
public void execute(QueueableContext context) {
// Logic to execute
System.debug(‘Queueable job is processing!’);
}
}
// Enqueuing the job
ID jobId = System.enqueueJob(new MyQueueableJob());
5. HttpCallout
Purpose: While not an interface in the traditional sense, using Http classes enables you to make HTTP requests to external services, essential for integrations.
Implementation: You create an instance of Http, configure the request, and send it.
Example:
public class HttpClient {
public HttpResponse makeGetRequest(String endpoint) {
Http http = new Http();
HttpRequest request = new HttpRequest();
request.setEndpoint(endpoint);
request.setMethod(‘GET’);
request.setHeader(‘Content-Type’, ‘application/json’); // Example header
HttpResponse response = http.send(request);
return response;
}
}
// Usage
HttpClient client = new HttpClient();
HttpResponse response = client.makeGetRequest(‘https://api.example.com/data’);
System.debug(response.getBody());
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Understanding and utilizing interfaces in Salesforce Apex can significantly enhance your code’s organization, reusability, and maintainability. By implementing various interfaces like Database.Batchable, Schedulable, and Queueable, you can leverage Apex’s capabilities to handle complex business logic efficiently. As you develop applications on the Salesforce platform, remember to apply best practices for interface design and implementation, ensuring a robust and scalable solution.