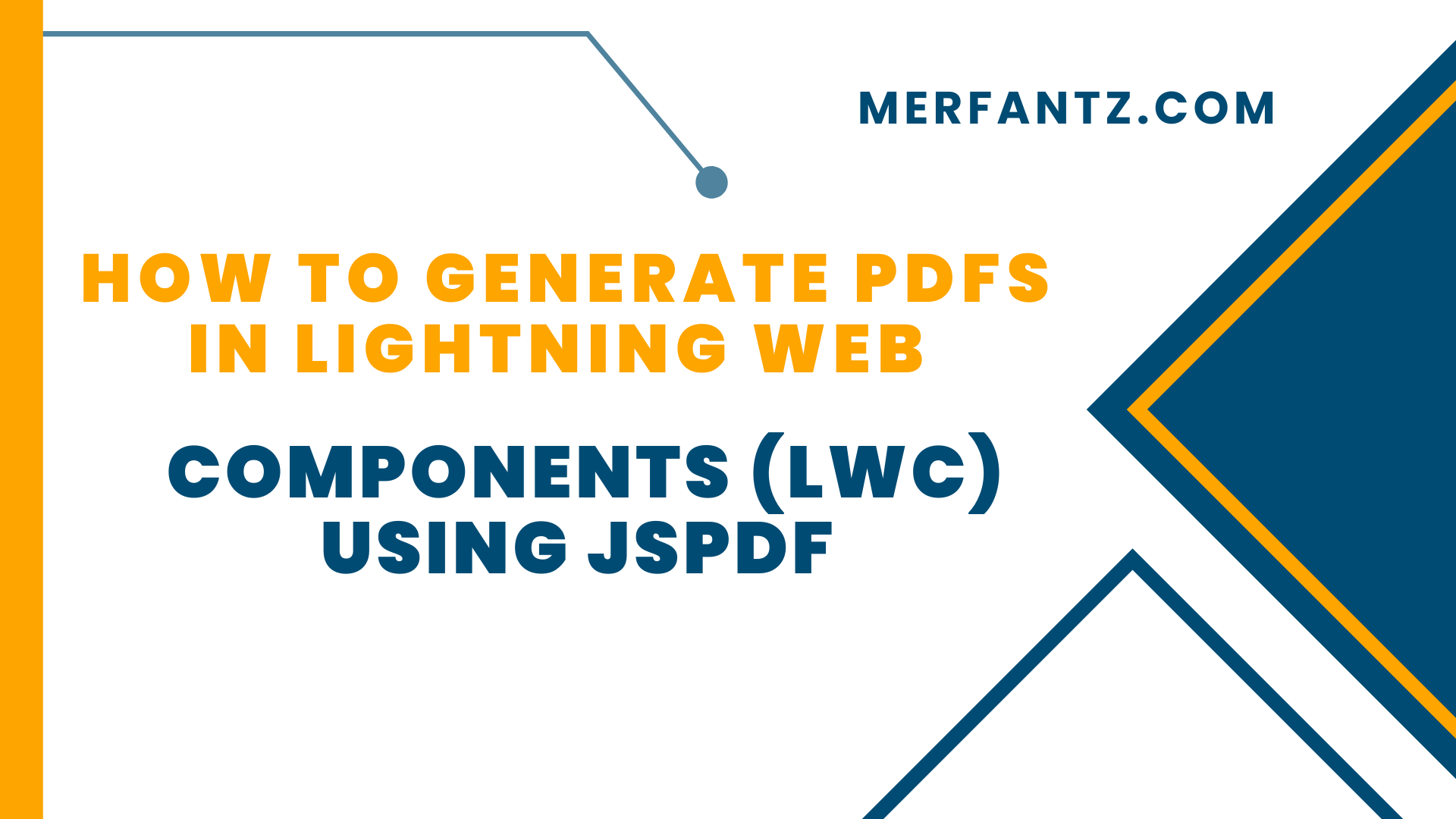
Introduction
When working with Salesforce, particularly in scenarios like generating invoices, reports, or contracts, the ability to generate PDFs dynamically is a powerful feature. In this post, we’ll explore how to use jsPDF, a popular JavaScript library, within Lightning Web Components (LWC) to generate PDFs directly from your Salesforce application.
What is jsPDF?
jsPDF is a client-side JavaScript library that allows you to generate PDF documents in the browser. It supports text, images, and various shapes, making it versatile for document creation. In the context of LWC, jsPDF can be used to create downloadable PDFs based on the data rendered on the page or dynamically from Apex.
Steps to Generate PDFs in LWC Using jsPDF
- Install jsPDF in Your Project
- Download the jsPDF library from the official jsPDF GitHub repository
- Go to Salesforce Setup and search for “Static Resources.”
- Click “New” and upload the jspdf.umd.min.js file. Name it jsPDF.
2. Create the LWC Component
Create a simple LWC component that will generate a PDF when a button is clicked.
HTML :
<template>
<lightning-card title=“PDF Generator”>
<div class=“slds-m-around_medium”>
<lightning-button label=“Generate PDF” onclick={generatePDF}></lightning-button>
</div>
</lightning-card>
</template>
JS :
import { LightningElement, api, track,wire } from ‘lwc’;
import { loadScript } from ‘lightning/platformResourceLoader’;
import jsPDFResource from ‘@salesforce/resourceUrl/jsPDF’;
import { NavigationMixin } from ‘lightning/navigation’;
import { getRecord } from ‘lightning/uiRecordApi’;
const fields = [‘Invoice__c.Customer_Name__c’,’Invoice__c.Name’,’Invoice__c.Product_name__c’,’Invoice__c.Quantity__c’,’Invoice__c.price__c’];
export default class GeneratePDF extends NavigationMixin(LightningElement) {
@api recordId;
InvoiceNo;
Customername;
Price;
Quantity;
Product;
jsPDFInitialized = false;
@wire(getRecord, {
recordId: “$recordId”,
fields: fields
})
invoiceData({
data,
error
}) {
if (data) {
console.log(‘data’ + JSON.stringify(data))
this.InvoiceNo = data.fields.Name.value;
this.Customername = data.fields.Customer_Name__c.value;
this.Price = data.fields.price__c.value;
this.Quantity = data.fields.Quantity__c.value;
this.Product = data.fields.Product_name__c.value;
} else if (error) {
console.log(‘Error value parse ‘ + JSON.stringify(error));
}
}
renderedCallback() {
if (!this.jsPDFInitialized) {
this.jsPDFInitialized = true;
loadScript(this, jsPDFResource).then(() => {
console.log(‘jsPDF library loaded successfully’);
}).catch((error) => {
console.log(‘Error loading jsPDF library’, error);
});
}
}
generatePDF() {
if (this.jsPDFInitialized) {
const { jsPDF } = window.jspdf;
const doc = new jsPDF();
// Title (centered and bold)
doc.setFontSize(18);
doc.setFont(“helvetica”, “bold”);
const title = ‘Invoice’;
const pageWidth = doc.internal.pageSize.width;
const titleWidth = doc.getTextWidth(title);
const xTitlePosition = (pageWidth – titleWidth) / 2;
doc.text(title, xTitlePosition, 10);
// Invoice Details (left-aligned)
doc.setFontSize(12);
doc.setFont(“helvetica”, “normal”);
doc.text(‘Invoice Date: ‘ + new Date().toLocaleDateString(), 10, 20);
doc.text(‘Customer:’+this.Customername, 10, 30);
// Table Header (bold)
doc.setFont(“helvetica”, “bold”);
doc.text(‘Invoice No’, 10, 50);
doc.text(‘Item’, 60, 50);
doc.text(‘Quantity’, 110, 50);
doc.text(‘Price’, 160, 50);
// Table Row (normal)
doc.setFont(“helvetica”, “normal”);
doc.text(”+this.InvoiceNo, 10, 60);
doc.text(”+this.Product, 60, 60);
doc.text(”+this.Quantity, 110, 60);
doc.text(‘$’+this.Price, 160, 60);
// Add Footer centered
const footer = ‘Thank you for your business!’;
const footerWidth = doc.getTextWidth(footer);
const xFooterPosition = (pageWidth – footerWidth) / 2;
doc.setFont(“helvetica”, “normal”);
const footerYPosition = 90; // Adjust this to ensure it’s below the last row
doc.text(footer, xFooterPosition, footerYPosition); // Position footer below the table
/// Convert the PDF into a Blob
const pdfOutput = doc.output(‘blob’);
// Create a URL for the Blob and open it in a new tab for preview
const blobUrl = URL.createObjectURL(pdfOutput);
window.open(blobUrl); // This opens the PDF in a new tab for preview
} else {
console.error(‘jsPDF is not initialized.’);
}
}
}
We have included a Lightning Button named ‘Generate PDF’. when clicked, it generates and downloads the PDF.
Output:
Using LWC and jsPDF, you could pull data from Salesforce objects (such as Opportunities, Orders, or Custom Objects) and generate a PDF on demand with a single click. Here’s how you could structure the component:
- Fetch data via Apex from Salesforce objects.
- Pass the data into jsPDF to populate dynamic content in the PDF.
- Download the customized PDF document with all the relevant details.
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Generating PDFs with jsPDF in LWC is a powerful way to create documents on the fly. Whether you need reports, invoices, or other documents, the combination of jsPDF and LWC allows for flexible and customizable solutions. With jsPDF’s extensive functionality, you can tailor your PDFs to suit your specific business needs in Salesforce.