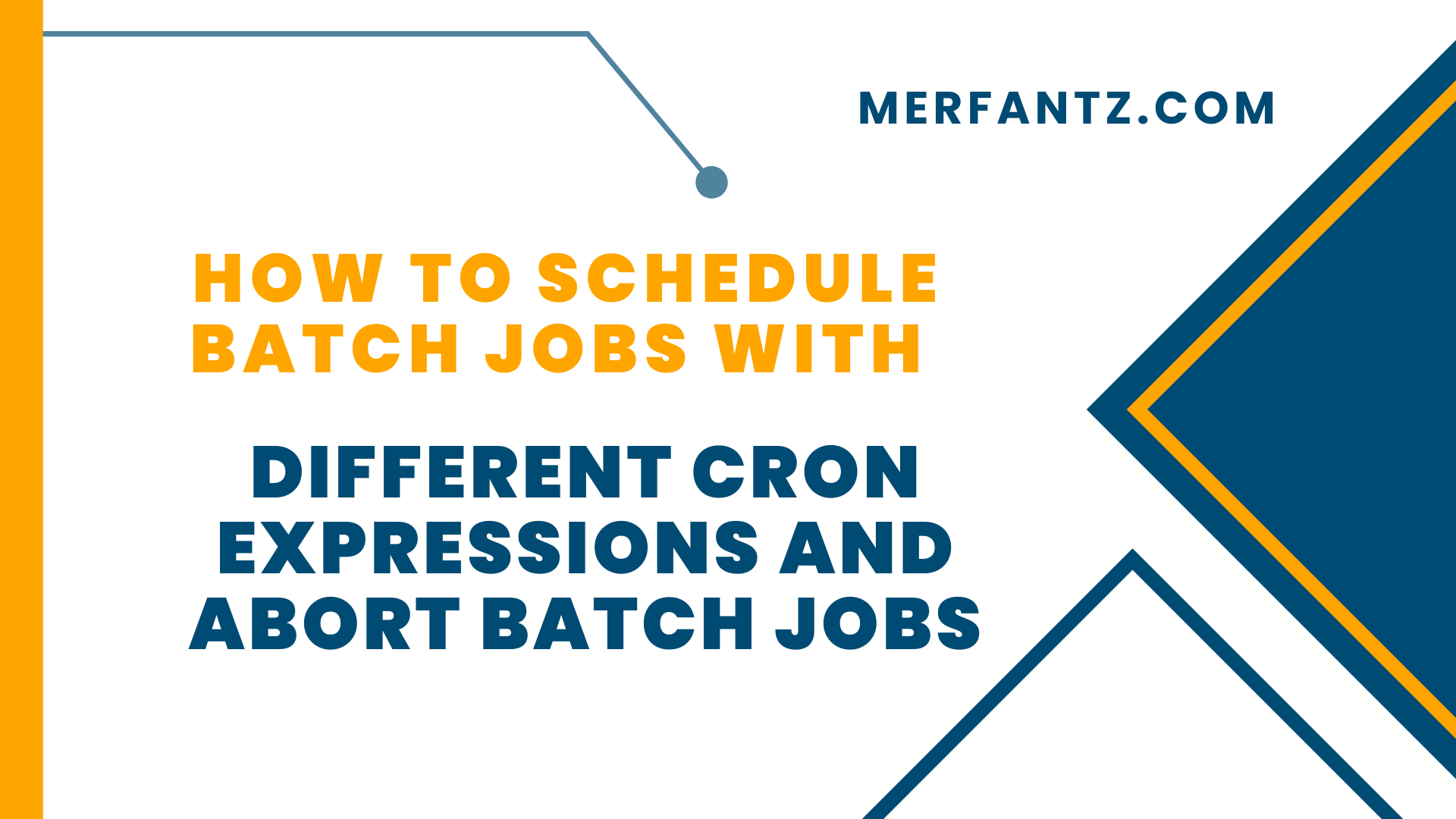
Salesforce provides robust tools for automating and processing large volumes of records asynchronously using batch jobs. One of the most powerful features is the ability to schedule these batch jobs to run at specific times using CRON expressions. Additionally, knowing how to abort running or scheduled batch jobs is crucial for maintaining control over your processes. This technical blog will explore how to schedule Salesforce batch jobs with different CRON expressions and how to abort them when necessary.
1.What Are CRON Expressions in Salesforce?
CRON expressions are strings used to define the schedule on which a job should be executed. Salesforce’s CRON syntax is a specialized version that includes fields for seconds, minutes, hours, day of the month, month, day of the week, and year.
Understanding the CRON Expression Fields
- Seconds (0-59): Always set to 0 because Salesforce does not support scheduling at the second level.
- Minutes (0-59): Specifies the exact minute when the job should run.
- Hours (0-23): Specifies the hour of the day (in 24-hour format).
- Day of the Month (1-31): Specifies the day of the month when the job should run.
- Month (1-12 or JAN-DEC): Specifies the month when the job should run.
- Day of the Week (1-7 or SUN-SAT): Specifies the day of the week when the job should run (Sunday is 1).
- Year (optional, 1970-2099): Specifies the year when the job should run (optional).
Examples of CRON Expressions:
- Every day at midnight: 0 0 0 * * ?
- Every Monday at 9 AM: 0 0 9 ? * 2
- First day of every month at 1 AM: 0 0 1 1 * ?
- Every day at 5 PM from January through March: 0 0 17 * 1-3 ?
Key Points to Remember:
- Use ? when you don’t want to specify a particular value, especially for Day of Month and Day of Week.
- Use * to match any value within the allowed range for the field.
2.How to Schedule Salesforce Batch Jobs with CRON Expressions
To schedule a batch job in Salesforce, you need to create a class that implements the Schedulable interface, alongside the Database .Batch able interface. Let’s walk through the process step-by-step.
Step 1: Create the Batch able Class
- The first step is to create a class that implements Database Batch able. This class contains three methods:
- start: Defines the query to fetch the records to be processed.
- execute: Contains the logic that processes each batch of records.
- finish: Defines any post-processing steps after all batches are processed.
public class MyBatchClass implements Database.Batchable<sObject> {
public Database.QueryLocator start(Database.BatchableContext bc) {
return Database.getQueryLocator([SELECT Id FROM Account WHERE BillingState = ‘CA’]);
}
public void execute(Database.BatchableContext bc, List<Account> scope) {
for(Account acc : scope) {
acc.BillingState = ‘NY’;
}
update scope;
}
public void finish(Database.BatchableContext bc) {
System.debug(‘Batch job completed successfully’);
}
}
Step 2: Create the Schedulable Class
Next, create a class that implements the Schedulable interface. This class will call the executeBatch method within the execute method.
public class MyBatchScheduler implements Schedulable {
public void execute(SchedulableContext sc) {
MyBatchClass batch = new MyBatchClass();
Database.executeBatch(batch);}}
Step 3: Schedule the Batch Job with a CRON Expression
Finally, schedule the batch job using a CRON expression by calling the System.schedule method. This can be done through the Developer Console or via anonymous Apex code.
String cronExpression = ‘0 0 1 1 * ?’; // At 1 AM on the 1st day of every month
MyBatchScheduler scheduler = new MyBatchScheduler();
System.schedule(‘Monthly Account Update’, cronExpression, scheduler);
3.Aborting Batch Jobs
Why Abort Batch Jobs?
- Discuss scenarios where you might need to abort a batch job, such as errors, changes in requirements, or performance issues.
Manually Abort Batch Jobs
Describe how to manually abort a batch job from the Salesforce UI:
- Go to Setup > Apex Jobs.
- Find the job you want to abort.
- Click Abort.
Programmatically Abort Batch Jobs
- Provide code to abort a batch job programmatically:
List<AsyncApexJob> jobs = [SELECT Id, Status FROM AsyncApexJob WHERE JobType = ‘BatchApex’ AND Status = ‘Processing’];
for (AsyncApexJob job : jobs) {
System.abortJob(job.Id);
}
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion
Effective scheduling of batch jobs using CRON expressions can help automate processes and manage large datasets efficiently in Salesforce. Additionally, knowing how to abort jobs when necessary can help in managing system resources and handling unexpected issues.