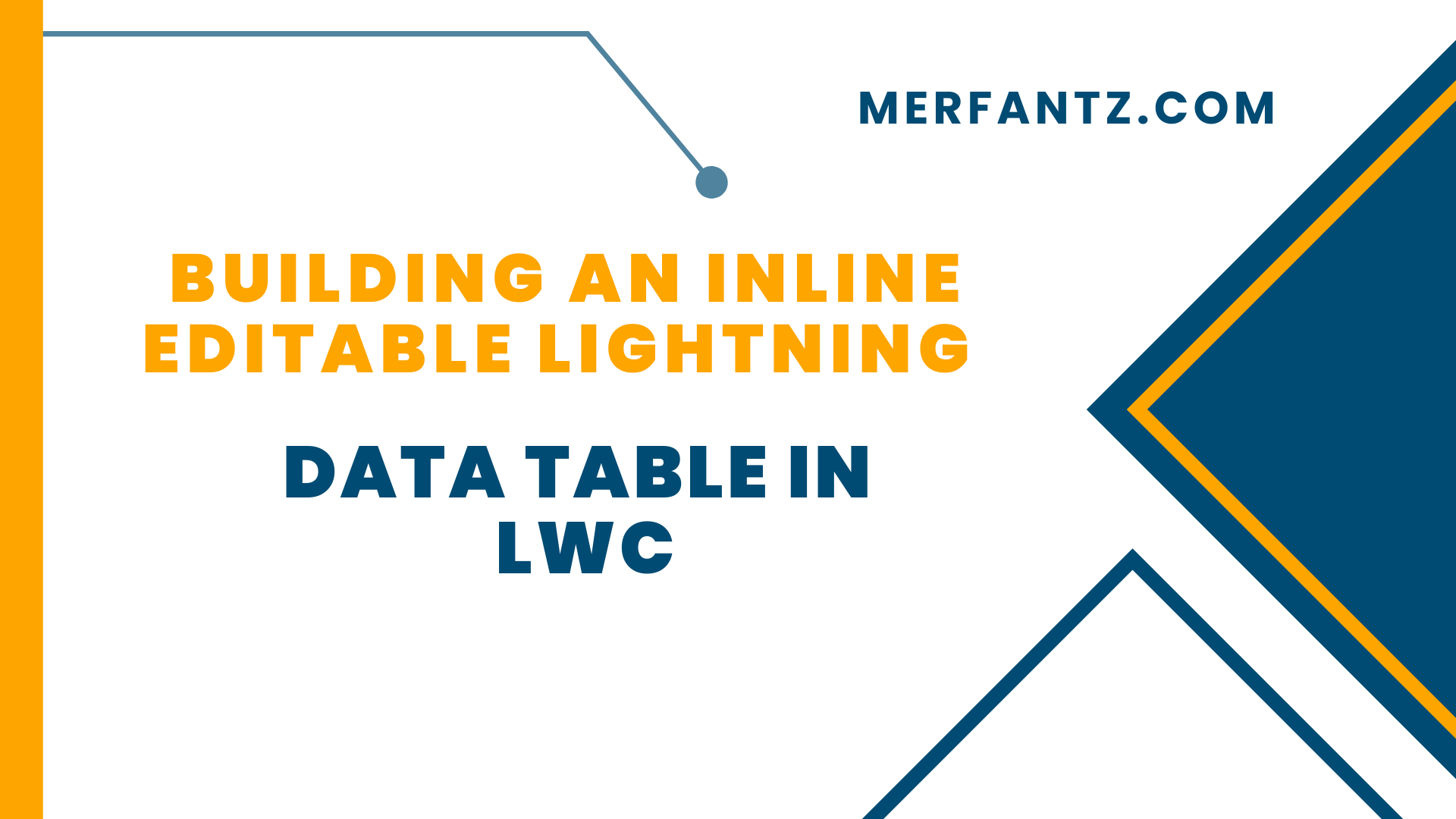
Introduction :
In this blog, we’ll walk you through the process of creating an inline editable Lightning Data Table in a Lightning Web Component (LWC). Inline editing is a powerful feature that allows users to edit records directly within the table without navigating to a different page or modal. By the end of this tutorial, you’ll have a functional Lightning Data Table that fetches contact records based on user input, allows inline editing, and updates the records in Salesforce.
Start by creating the HTML template for our LWC. The template consists of a lightning-card that contains a search input and a lightning-datatable component.
Here is the code below.
<template>
<lightning-card title=”Search Contacts” icon-name=”custom:custom63″>
<div class=”slds-m-around_medium”>
<lightning-input
type=”search”
onchange={handleKeyChange}
class=”slds-m-bottom_small”
label=”Search”>
</lightning-input>
<template if:true={contacts}>
<div style=”height: 300px;”>
<lightning-datatable
key-field=”Id”
data={contacts}
columns={columns}
hide-checkbox-column=”true”
show-row-number-column=”true”
onsave={handleSave}
draft-values={draftValues}>
</lightning-datatable>
</div>
</template>
<template if:true={error}>
{error}>
</template>
</div>
</lightning-card>
</template>
In this template:
- lightning-input: Captures the search input from the user.
- lightning-datatable: Displays the contact records in a table format, with inline editing enabled on specific columns.
Now, let’s move on to the JavaScript controller where we’ll handle the data fetching, inline editing, and updating logic.
import { LightningElement, track } from ‘lwc’;
import getContacts from ‘@salesforce/apex/LWCDataTableExample.getContacts’;
import { updateRecord } from ‘lightning/uiRecordApi’;
import { refreshApex } from ‘@salesforce/apex’;
import { ShowToastEvent } from ‘lightning/platformShowToastEvent’;
const columns = [
{ label: ‘Id’, fieldName: ‘Id’ },
{ label: ‘First Name’, fieldName: ‘FirstName’, editable: true },
{ label: ‘Last Name’, fieldName: ‘LastName’, editable: true }
];
export default class LwcLightningDataTableDemo extends LightningElement {
@track contacts;
@track error;
@track columns = columns;
@track draftValues = [];
handleKeyChange(event) {
const strLastName = event.target.value;
if (strLastName) {
getContacts({ strLastName })
.then(result => {
this.contacts = result;
})
.catch(error => {
this.error = error;
});
} else {
this.contacts = undefined;
}
}
handleSave(event) {
const recordInputs = event.detail.draftValues.slice().map(draft => {
const fields = Object.assign({}, draft);
return { fields };
});
const promises = recordInputs.map(recordInput => updateRecord(recordInput));
Promise.all(promises).then(contacts => {
this.dispatchEvent(
new ShowToastEvent({
title: ‘Success’,
message: ‘All Contacts updated’,
variant: ‘success’
})
);
this.draftValues = []; // Clear draft values
return refreshApex(this.contacts); // Refresh the datatable with updated data
}).catch(error => {
this.dispatchEvent(
new ShowToastEvent({
title: ‘Error’,
message: ‘An error occurred while updating records’,
variant: ‘error’
})
);
});
}
}
Explanation of the JavaScript Controller:
- @track: Used to track changes in properties that the template relies on. Here, we track contacts, error, columns, and draftValues.
- handleKeyChange: This method is triggered when the user types in the search input. It calls an Apex method getContacts with the entered search term and updates the contacts property with the result.
- handleSave: This method handles the save action when the user makes inline edits. It maps the draft values to record inputs, calls updateRecord to save the changes in Salesforce, and then refreshes the datatable to display the updated data.Here’s the XML configuration:<?xml version=”1.0″ encoding=”UTF-8″?>
<LightningComponentBundle xmlns=”http://soap.sforce.com/2006/04/metadata” fqn=”lwcLightningDataTableDemo”>
<apiVersion>47.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__HomePage</target>
<target>lightning__Tab</target>
</targets>
</LightningComponentBundle>
See how FieldAx can transform your Field Operations.
Try it today! Book Demo
You are one click away from your customized FieldAx Demo!
Conclusion :
we’ve created a Lightning Data Table with inline editing functionality using LWC. This feature enhances user experience by allowing quick edits directly within the table, without needing to navigate away from the page. You can extend this component further by adding additional columns, incorporating more complex logic, or enhancing error handling.